In this post, we will provide a Simple Java JMS Client Program to Test Active MQ Queue and Topic, this program is designed to take username and password(securely) and the broker URL from the user at the runtime.
It is designed to do both Send and Receive operations for both types of message destinations (Queue and Topic).
To be more clear, this tool is to
- Publish and subscribe to the ActiveMQ Topic
- Produce and consume messages from the ActiveMQ Queue ( one by one and all at once)
- In subscription, this program offers two methods for the user
- Message Listener Subscription
- Durable Subscription
Environment Setup for ActiveMQ
If you are already having a working ActiveMQ installation. It's Great.!. If not, I would recommend you to read the following article Active MQ Installation, Security Setup, and Hardening - How to
The preceding article covers both installation and Security Hardening like Web console Security, Securing the Broker, Enabling Role-based Access to the Queue and Topics (Authorization) and Usage of Encrypted Passwords. That is more than enough for you to get started with ActiveMQ. Good Luck!
Let us consider that you are now having a working ActiveMQ setup and move on.
The ActiveMQ version used in this post is ActiveMQ-5.15.3
Note*: For user convenience, we have wrapped all the class files into a JAR, which can be used as Command Line-based tool to process the messages from both ActiveMQ Queue and Topic. We call it AMQCLI.jar
It has various features included like
|
If you have come here looking for just the code. click in this link to go to our GitHub repo and download the code.
How to use the Simple JMS Client - AMQCLI.jar
Let us see how to use AMQCLI for various operations like sending and receiving messages to the queue and publishing and subscribing to the topic.
As a first step, Download the AMQCLI.jar file and keep it in your desired local system path
An AMQCLI is a Java Program and a CLI based tool to interact with ActiveMQ Queue and Topic and Process the Messages. You can read and send messages, You can publish and receive messages with AMQCLI.
It also has been bundled with many other cool features. Let's Go and Explore
How to Send Message to the Queue
Launch the AMQCLI.jar to send the message to the Queue. as you could see, it is designed to get all the details from the user at runtime and reads the password securely.
$ java -cp AMQCLI.jar QueueSender
-------------------------------
ACTIVEMQ - QUEUE SENDER - CLI
-------------------------------
Enter the Queue Name (or) quit/Quit to Exit [] :TestQueue
Enter the details to Connect to ActiveMQ
Enter the UserName : admin
Enter the Password :
Enter the ConnectionURL : tcp://localhost:61616
Enter the message to send:
Hello there
INFO | sent message with text='Hello there'
Enter the Queue Name (or) quit/Quit to Exit [TestQueue] :
INFO | Taking the Already Entered QueueName - TestQueue
INFO | Taking Already Entered Username,Password and ActiveMQ URL
Enter the message to send:
This is testing
INFO | sent message with text='This is testing'
Enter the Queue Name (or) quit/Quit to Exit [TestQueue] :quit
You have entered Quit, We are not sure if that's Queue Name.
Please Clarify?
1 => Press One to Exit
2 => Press 2 to Reset & Continue
3 => Quit is my Topic Name
Your Option :1
How to Receive or Read the Message from the Queue
This is a Client Program to receive or read the messages from the Queue
While consuming messages, AMQCLI needs additional arguments to control whether ALL the messages should be read from the queue (or) just the first one.
In order to control this setting, you need to pass any one of the following value as an additional argument
- To receive only one message ( the oldest ) from the Queue, use
onebyone
- To Receive all the messages recursively from the Queue, use
allatonce
Let us see the examples of both.
Reading the messages OneByOne
$ java -cp AMQCLI.jar QueueReceiver onebyone
-------------------------------
ACTIVEMQ - QUEUE RECEIVER- CLI
-------------------------------
Enter the Queue Name (or) quit/Quit to Exit [] :TestQueue
Enter the details to Connect to ActiveMQ
Enter the UserName : admin
Enter the Password :
Enter the ConnectionURL : tcp://localhost:61616
INFO |
received message with text='Hello'
INFO | message acknowledged
INFO | Hello
Enter the Queue Name (or) quit/Quit to Exit [TestQueue] :
INFO | Taking the Already Entered QueueName - TestQueue
INFO | Taking Already Entered Username,Password and ActiveMQ URL
INFO |
received message with text='Hello there'
INFO | message acknowledged
INFO | Hello there
Enter the Queue Name (or) quit/Quit to Exit [TestQueue] :
INFO | Taking the Already Entered QueueName - TestQueue
INFO | Taking Already Entered Username,Password and ActiveMQ URL
INFO |
received message with text='This is testing'
INFO | message acknowledged
INFO | This is testing
Enter the Queue Name (or) quit/Quit to Exit [TestQueue] :quit
You have entered Quit, We are not sure if that's Queue Name.
Please Clarify?
1 => Press One to Exit
2 => Press 2 to Reset & Continue
3 => Quit is my Topic Name
Your Option :1
[email protected]:/users/aksarav/NetBeansProjects/Messaging/dist$
Reading the messages All at once
$ java -cp AMQCLI.jar QueueReceiver allatonce
-------------------------------
ACTIVEMQ - QUEUE RECEIVER- CLI
-------------------------------
Enter the Queue Name (or) quit/Quit to Exit [] :TestQueue
Enter the details to Connect to ActiveMQ
Enter the UserName : admin
Enter the Password :
Enter the ConnectionURL : tcp://localhost:61616
INFO | received message with text='Hello'
INFO | message acknowledged
INFO | Hello
INFO | received message with text='Hello there'
INFO | message acknowledged
INFO | Hello there
INFO | received message with text='This is testing'
INFO | message acknowledged
INFO | This is testing
INFO | no more messages to read from the queue
Enter the Queue Name (or) quit/Quit to Exit [TestQueue] :quit
You have entered Quit, We are not sure if that's Queue Name.
Please Clarify?
1 => Press One to Exit
2 => Press 2 to Reset & Continue
3 => Quit is my Topic Name
Your Option :1
Let us continue to see how AMQCLI works with Topics and Subscriptions
We will see how the two models of subscription works. First, we will test the Message Listener Model
How to Subscribe to the Topic using the Message Listener model [Non-Durable]
As the Message Listener model is not Durable, the subscriber has to be online when the message is published. Once the subscriber is disconnected /exited from the session. ActiveMQ will forget the subscriber and even if it comes online again. the messages pending in the Topic would not be delivered.
In order to test this model, I will have two parallel terminals and launch AMQCLI to publish and to subscribe at the same time.
Something like shown below. As you could see. I have two parallel terminals. First I initiate the subscriber (on the left) session with the following command
java -cp AMQCLI.jar TopicCLI subscribe
Note* Subscriber session would last for 5 minutes and wait for messages as its being sent to the Topic. It is the Synchronous Messaging model.
It uses the OnMessage JMS method to listen to the Topic, in other words, the Onmessage method gets invoked as the new message arrives at the Topic.
This makes it clear that we cannot receive the pending messages in the Queue with this type of model
Second, I will initiate the publisher session by the following command to send some messages to the Topic.
java -cp AMQCLI.jar TopicCLI publish
The following snapshot would give you a quick insight on how it looks like to publish and subscribe the messages.
As you are initiating the subscribe
session, there will be a new Non-Durable subscriber created
with the client ID MessageListener-TopicCLI
.
How to view the Subscriber in ActiveMQ Web Console
If you would like to verify it, you can log in to the ActiveMQ web console and go to subscribers tab and see it yourself
as you quit/close the subscription session in AMQCLI. These entries would no longer be there in the web console.
How to Subscribe to the Topic using the Subscriber and Consumer model [ Durable ]
This is a sample client program to subscribe to the ActiveMQ Topic.
This is an asynchronous messaging model, where the subscriber does not have to be available online to receive the message when the message is being sent.
The messages arrived at the time when the Subscriber is not ACTIVE/ONLINE. JMS provider (ActiveMQ) would retain the message until the subscriber comes online and reads the message (or) until the message gets expired. In other words, this is called Message Persistence.
Note*: JMS provider's message retention period for a message is controlled by the expiry date/time configuration of each individual message.
To create a durable subscription, you must provide two unique identifiers during the subscriber initialization. From then you can use the same identifiers to re-connect to your durable subscription session and to receive the pending message that arrived at the topic when you ( subscriber) were offline.
The identifiers are
- Subscriber Name
- Consumer Name
Caution*: If there is any simple typo in these identifiers. you would be considered as a new subscriber and you cannot receive the pending messages on the topic. |
To Create a Durable Subscription using AMQCLI use the following command
java p AMQCLI.jar TopicCLI durable
In order to test the Durable subscription with AMQCLI. Follow the below steps
- Establish a Publisher session with AMQCLI to
TestTopic
( choose the Topic as you desire ) and send one message - Now create a durable subscriber with AMQCLI and target it to the Same
TestTopic
- Enter the
SubscriberName
andConsumerName
as you wish and make sure you remember it - Close the Durable Subscriber session
- Go to the ActiveMQ web console and go to the subscribers page and verify that the subscriber you have created in Step3 is available under
Offline Durable Topic Subscribers table
- If the Publisher session, we created in Step1, is active. use the same (or) create a new Publisher session and send some messages to the
TestTopic
[ Note that the durable subscriber is offline as we sending this messages ] - Repeat the steps 2 and 4 and use the same
SubscriberName
andConsumerName
you have provided in Step 3 - Now you will receive the messages published/sent in Step 5 when the durable subscriber was INACTIVE/OFFLINE
Screenshots of those steps being performed ( Click to Enlarge )
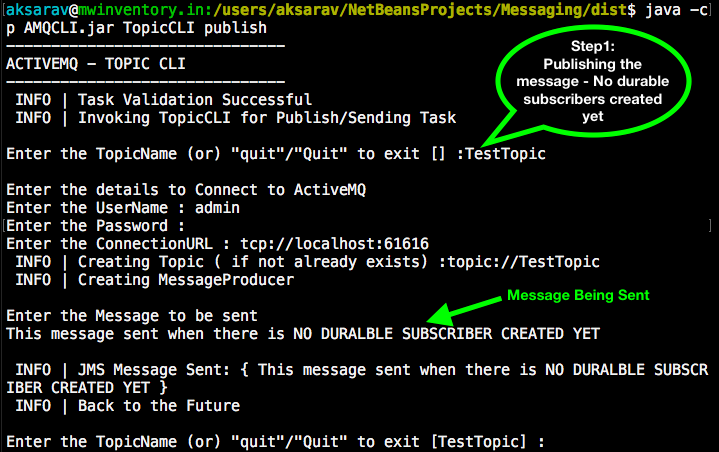
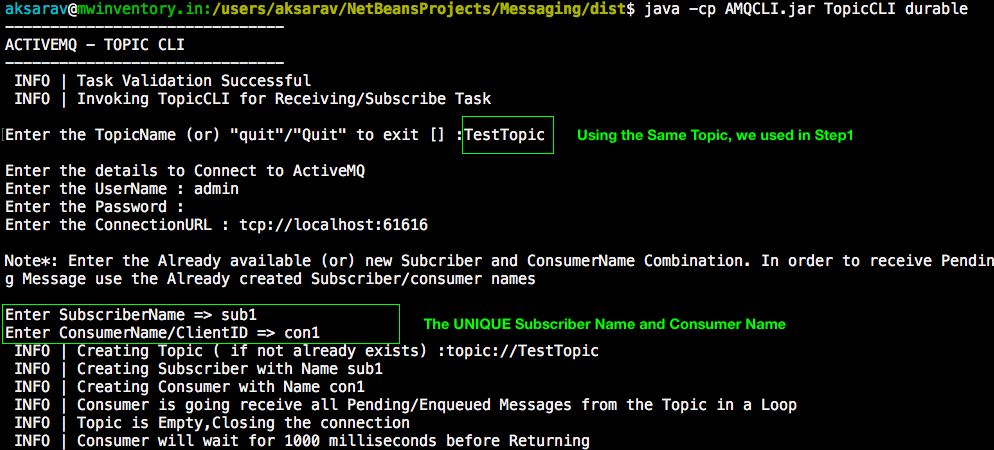

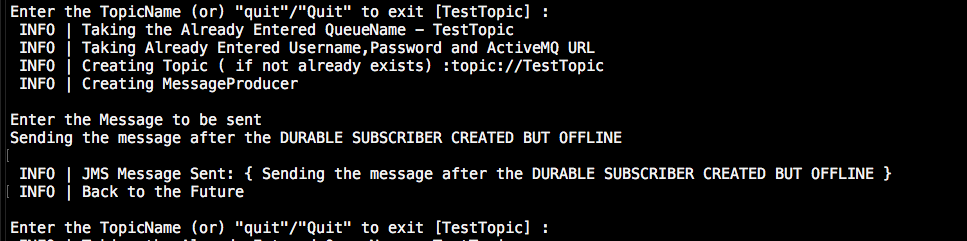
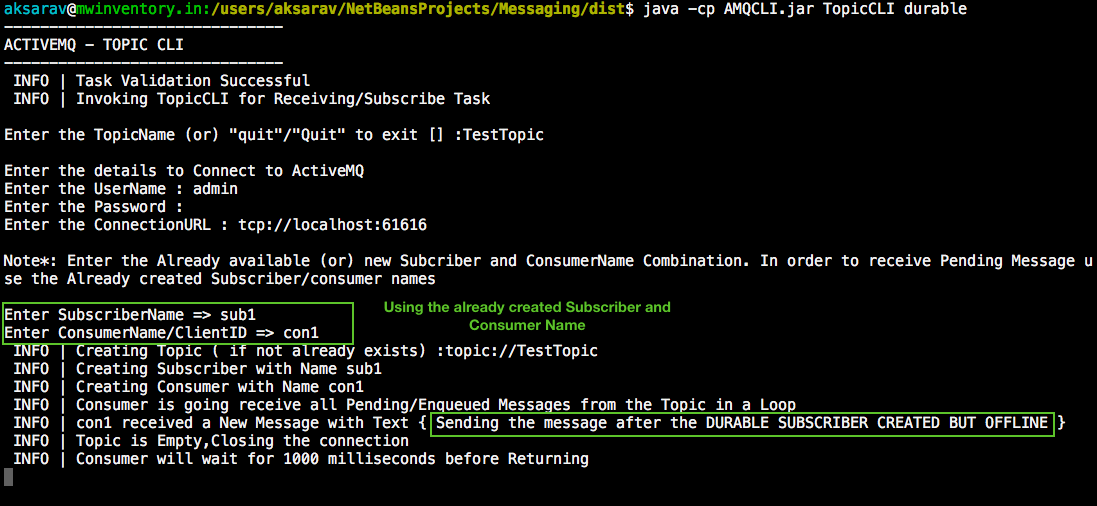
Hope this post helps
Please post your comments and feedback
Rate this article [ratings]
Cheers,
Sarav AK
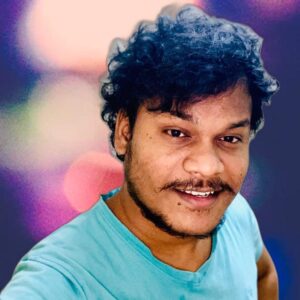
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content