When you are using CURL the problem is that sometimes you would have to compromise on security as it requires you to keep the passwords in clear text. But there is a way we can avoid it and this is post is about
"How to Avoid or Hide Sending Clear Text username and Password to CURL? "
Well, This post introduces an efficient and secure way to pass username and password to CURL while making REST API requests which requires BASIC Authentication.
SecureCLI encrypts the username and password after collecting them from the user over the prompt and reuses it as long as the Session of the program execution is LIVE, in other words until you terminate the script.
You can enter username and password only once and make so many subsequent REST API calls.
This is a Python-based solution and I presume that your Linux system has Python. ( Well All the latest Linux systems have python pre-installed).
If you are trying to login to an HTTPS URL with TLS/SSL security. you might need openssl library installed in your system as well.
Since this is a wrapper script over CURL. this uses CURL command to do the actual work. so you should have all these three dependencies before using the secure CLI.
with no further ado. let's jump into the objective.
How to avoid cleartext password in CURL - Secure CURL
These are the steps to implement Secure CURL script. Which is written in Python and would come handy when you are using CURL next time and sending the password as a cleartext.
Step1: Copy the script displayed below and save it in your Server with .py extension
#!/usr/bin/python
#
#
# Author: Sarav([email protected])
# Name: SecureCLI-Curl.py
# Date: 27-March-2018
#
#
import os
import sys
import getpass
import time
import base64
import subprocess
import re
text=[]
output = ""
print "-"*90
print "\t\t Secure CLI for CURL - No more clear text password"
print "-"*90
print "Instrutions\n------------"
print "This is Secure CLI for CURL tool created to efficiently pass the username and password to CURL"
print "Now you will be prompted for username & password"
print "After Entering the username and password you DO NOT have to use – user username:password in your request header"
print "The Tool will take care of passing the credentials as a Authorization header"
print "Note*: Your details will not be saved and only valid for this session"
print "\nExample:"
print "--------"
print " curl -v \ "
print " -H X-Requested-By:MyClient \ "
print " -H Accept:application/json \ "
print " -X GET http://localhost:7001/management/weblogic/latest/serverConfig?links=none "
print " END"
print "-"*90
# Get username and password
username = raw_input("Enter the Username: ")
password=getpass.getpass("Enter the Password: ")
cred_enc=base64.b64encode(username+":"+password)
print "INFO: the entered user credentials have been encrypted and will be sent as a header"
#cred_dec=base64.b64decode(cred_enc)
#print cred_dec
def makerequest():
print ""
print "Enter the whole request line by line, type END and hit enter once done"
#read -d '' i << END
while True:
line=raw_input("")
text.append(line)
if "END" in line:
break
text.pop()
#update the basic auth header
text.insert(1,'-H "Authorization: Basic '+cred_enc+'"')
cmd = " ".join(text)
cmd=re.sub('[\\\]','', cmd)
print "=========================="
print "Execution Result"
print "=========================="
print ""
output = subprocess.Popen([cmd], shell=True, stdout = subprocess.PIPE).communicate()[0]
print output
print ""
print "=========================="
makerequest()
while True:
ans=raw_input("Would you like to Proceed and Make a New Request ? Yes to Continue| No to exit : ")
if "y" in ans or "Y" in ans or "yes" in ans or "Yes" in ans or "YES" in ans:
text=[];
makerequest()
else:
sys.exit(0);
Step2: Make sure it is executable (in unix you can give Executable to all by issuing "chmod a+x SecureCLI-Curl.py" )
Step3: Execute it as follows.
python SecureCLI-Curl.py (or) ./SecureCLI-Curl.py
Enter the username and Password and then make a CURL call as usual. Except that you do not have to use the – user (or) -u flag or enter clear text password. The SecureCLI will take care of encrypting your username and password into the Base64 format and appends it to the Authorization Request Header
Execution Result
Some Clarifications:
- You can use all the flags your general CURL supports like -k, – insecure – silent – ssl --user-agent etc.
- SecureCURL does not save any username and password anywhere
- This is Session Oriented when you re-execute the script you will be prompted to enter user credentials again.
- While entering the request, you do not have to give backslash \ if you are giving, The SecureCLI will trim and remove it
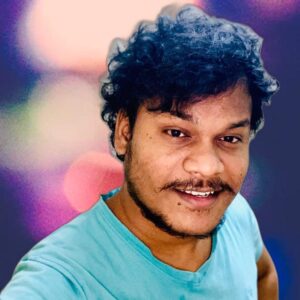
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content