In this post, we are going to briefly cover, What is web services and its types? How to develop a Simple SOAP Web service for Weblogic? followed by deployment and testing using POSTMAN and SOAP UI
We use Weblogic 12c application server as a platform to deploy our Simple SOAP Web service application and it is designed using the javax.jws
package and its @WebService and @WebMethod annotations.
The post also contains the Netbeans Project setup instructions and code explanations along with downloadable War file to practice on your own.
All the way down, we have given self-explanatory images and clip arts to make this more appealing. We have also provided screenshots and video clips where ever possible and needed. Hope you would enjoy this.
Let's get started.
Topic Index
- What is Web Service?
- Where, web services are used?
- What are the Types of Web Service?
- The Sample SOAP web service application?
- Netbeans Project Setup
- Code Explanation
- Initial Validation using Web Browser
- Real-time Testing with SOAPUI and POSTMAN
- Download Location for Code and War file
- Conclusion
What is WebService?
Put simply, Webservice is a medium to establish a communication between two cross-platform devices (or) programs commonly known as client and server over the world wide web.
In other words, Webservice can be defined as
A standard for enabling a communication between two programs and devices.
- A Method or technology for two devices to communicate over the internet (interoperable)
- The system that facilitates the communication between cross-platform devices or applications Like PHP to .NET to JAVA etc.
- Provides a specific service and directly perform the logic without any presentation (or) interface.
- Provides an operation (or) data as a service.
For example, Let's say you want to book a ticket in IRCTC Tatkal and instead of navigating to multiple screens and entering the details and captchas.
Would it be nice for you to be able to send a request in some format (like XML) with your username and password (or) may be an Authentication Token along with your train selection to IRCTC and getting your ticket booked in seconds?
Well, that's WebService for you.!.
Where, Web Services are being used?
Webservice is widely used across all sectors in IT such as Retail, Banking, E-Commerce, Stock Management, Inventory Management, Domain registration, Flight booking, Media.
As a real-time usage.
- An e-commerce based organization Amazon could use web service for automatic Inventory management and stock management and automate the process of item procuring and tracking.
- Hotel booking application booking.com could use web services to communicate to the various hotel vendors and be able to connect to their cross-technology systems and make a booking.
- Flight booking website makemytrip.com could use web services to talk to various service providers like Indigo, TruJet, AirIndia, SpiceJet and make someone fly
So on and So forth.
What is the difference between web application and web service?
A web application is a software application that a user runs in the web browser. A web service is an Application Program Interface (API) that runs on the server, which provides data to the client over HTTP through a standardized messaging system. (XML, JSON, etc...).
What are the Types Of Web Services?
Based on the standards and the technology being used, Web Services are categorized into two major types they are as follows.
SOAP Web service
- A Webservice implemented using SOAP Simple Object Access Protocol and XML are called as SOAP web services.
- It was the first and widely used webservice in the world, SOAP web services are being used for a long period of time, even before REST web service was conceptualized.
- It has been primarily designed to enable communication between programs written on different platforms and services. ( JAVA to .NET, .NET to PHP etc)
- It strictly follows the XML WSDL (Webservice Description Language) specifications and supports XML format only, No other formats like HTML/JSON is supported
RESTful Web service
- A Webservice conform(adhere) to the REpresentational State Transfer architecture is called as RESTful web services.
- It's built on top of normal web resources available on World wide web, commonly identified by the URL - Uniform Resource Locator
- It uses HTTP Protocol and with a payload(data) formatted in either of
HTML, JSON, XML
- Since it uses HTTP, It can support all the CRUD methods and the standard
GET, POST, OPTION, PUT, DELETE
- Fast performance and Less bandwidth
- Easy to implement. Unlike SOAP Web service, we can invoke the RESTful Web service using our normal web browser.
The Sample SOAP WebService Application
To comply with the ultimate purpose of this post and to demonstrate how the SOAP-based Web services are working we have created a Simple SOAP web service application using JAX-WS
of Java
We named the application as TestWebService
The "TestWebservice" Application is designed with following features/key factors.
- A Servlet program/interface to display the following information of the request ( more like Snoop Servlet)
- Request URL
- Request Headers
- URL the request has been made to
- Cookies
- Request Method ( GET/POST/OPTIONS/PUT) etc
- A SOAP webservice with two methods
- hello - To Say Hello the name we are passing in the request
- GetRhymingWord - Extract the word in the request and send to External API to get the words list that sounds similar to what was given. For example, If the user send "test" to the server as the word the output will be return with words like "rest" "mist" etc.
GetRhymingword method uses the normal Java HTTPURLConnection class and invokes the external API https://api.datamuse.com to find the list of Rhyming words which sound similar to the requested word.
Netbeans Project Setup
For further development purposes and for the better understanding of the application from a development perspective. I am going to explain the project setup of this application in Netbeans.
You can download the Netbeans ZIP version of this project from here
Code Explanation
Note*: For better page readability and loading time, we have not pasted the full code here. you can find each of these files in the GITHUB page of this project
The Snoop Servlet Gateway.java
It is a general servlet, Accepts the user request and renders the JSP page named welcome.jsp
RequestDispatcher view = request.getRequestDispatcher("welcome.jsp");
code snippet to render the JSP file is given above
The Frontend welcome.jsp
The JSP file is designed to get the request related information using the methods of request object and display the results in a table.
<table> <% Enumeration enumeration = request.getHeaderNames(); while (enumeration.hasMoreElements()) { String name=(String) enumeration.nextElement(); String value = request.getHeader(name); %> <tr> <td> <%=name %> </td> <td> <%=value %> </td> </tr> <% } %> </table>
The Webservice WS.java
This is normal Java file and uses javax.jws JAX-WS
of Java and its @WebService and @WebMethod annotations to create the webservice
and web methods.
A pretty simple code which speaks for itself. The Complete content of WS.java
is given below
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package com.middlewareinventory; import java.io.IOException; import javax.jws.WebService; import javax.jws.WebMethod; import javax.jws.WebParam; /** * * @author aksarav */ @WebService(serviceName = "WS") public class WS { /** * This is a sample web service operation */ @WebMethod(operationName = "hello") public String hello(@WebParam(name = "name") String txt) { return "Hello " + txt + " ! Welcome to middlewareinventory"; } /** * Web service operation */ @WebMethod(operationName = "GetRhymingWord") public String GetRhymingWord(@WebParam(name = "word") String word) throws IOException { //TODO write your implementation code here: HTTPCaller test = new HTTPCaller(word); String output = test.sendGET(); return output; } }
The HTTP Caller class to invoke external REST API HTTPCaller.java
If you glance once again at the WS.java
you could notice the following line, under the GetRhymingWord method implementation
HTTPCaller test = new HTTPCaller(word);
as you can see, it actually invokes the HTTPCaller Object and passes a word as an argument for which we need to find rhyming words for.
When HTTPCaller gets the word, It passes the word as Query String to the External REST API as follows
https://api.datamuse.com/words?rel_rhy=<word to search>
The HTTPCaller constructor code which is responsible to invoke the External API is given below
public HTTPCaller(String word) throws IOException { wordtosearch = word; System.out.println(" Wordtosearch " + wordtosearch ); GET_URL = "https://api.datamuse.com/words?rel_rhy=".concat(wordtosearch); }
Having the Code Explanation part completed. Let us go straight into deployment and testing
My Infrastructure Overview
Before we proceed further to test the application let us take a quick look at my Environment (or)infrastructure details being used for this post.
Application Server: Weblogic 12c Application Server IP : 192.168.60.4 Application Server Port: 18001 Application Name: TestWebservice
The "TestWebService" Application is already deployed into my local weblogic and ready for us to test. All the Example URLs going to be used in this post will refer to the infrastructure details presented above.
Initial Validation
Testing the Snoop Servlet
Snoop Servlet URL: http://192.168.60.4:18001/TestWebService/
In the following screenshot, you can notice that the page is displaying all the request related information including Header and Cookies
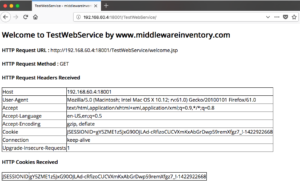
Testing the Webservice
Web Service URL: http://192.168.60.4:18001/TestWebService/WS
In the following image shows the help page of web service when being accessed over the Web browser. It contains the WSDL url for the Web Service.
The WSDL Page
WSDL page URL: http://192.168.60.4:18001/TestWebService/WS?wsdl
WSDL is an abbreviation of Web Service Description Language which tells the client about the methods available in the webservice and how to invoke them. Refer the following image shows the WSDL of our Web Service.
The Real-Time Testing
Having the application already deployed in weblogic. We are all set to move ahead and validate and our web service. Since it is a SOAP Web service. we need a special Client to invoke the web services.
I have two famous products in mind. One is the ultimate SOAP-UI another one is Google's POSTMAN
Most of you might raise your eyebrows by now and ask me "You sure? Postman ?" The answer is. Yes, am very sure.
Don't underestimate the power of common man and Postman
SOAP UI
Create a new SOAP Project using the WSDL of our web service http://192.168.60.4:18001/TestWebService/WS?wsdl
using the menu
File -> New Soap Project
Once the project is created you would be able to see the web methods available in our web service in the projects window of SOAP UI as shown below
If you click on the request you will be having a sample payload ( request XML) as shown below
Now replace the question mark
'?' with the word of your choice and submit the request
Now see the response contains the rhyming word list of Magic
. In the preceding Image, you could see 'Pelagic
' and 'tragic
'
Here is a vlog of me performing the SOAP UI testing. In video, I have also explained the codes and other various elements of this project. I would recommend you to see the video for more detailed information and understanding
Screen recording (VLOG)
THE GOOGLE POSTMAN
you could do the same thing with POSTMAN also. Only difference is that you should be aware of the SOAP syntax and payload information to pass.
Now I have copied the payload (request XML) created and tested in SOAP UI to invoke the web method or web service in POSTMAN
Am I the only one who feels the POSTMAN is more colourful and neat here?
Thereby, We have successfully invoked the GetRhymingWord
web service (or) web method successfully from both SOAP UI and POSTMAN
We Made it. !
Download Location for Code and War file
Netbeans exported ZIP file and WAR file has been uploaded into the following repository in GITHUB
https://github.com/AKSarav/SampleWebService
Conclusion
Hope this post was helpful and informative. For any queries and feedback please free to comment (or) directly mail me at [email protected]
Thanks to datamuse for providing such a wonderful API for free, which has been used as external REST API in GetRhymingWord
Method of our TestWebservice.
Cheers,
Sarav AK
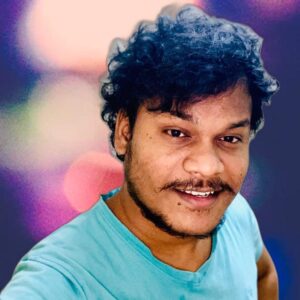
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content