In this article, I am going to share a shell script to help you get the TTL (time to live) and Memory Usage of all the keys available in Redis DB
Every tool/technology that we use in infrastructure comes with a certain cost of management.
Redis, a memory-intensive caching solution is no exception.
If we are not keeping track of what we are storing in Redis and how long we are storing it. Redis Memory usage will spike and it can bring your Production infrastructure down.
It can also burn your pocket and increase the Infrastructure costs. So it is always recommended to keep track of any data storage.
Whether it is long storage like DB or short storage like Redis Cache.
Now, To the objective.
A solution to get TTL and Memory Usage of all the keys in Redis Database
I hope we are on the same page that how necessary it is to monitor the Redis database Keys and their size.
Of course, there are various solutions and integrated monitoring systems to monitor the TTL with configurable threshold alerts.
Now we are going to see a quick and easy solution to get TTL and Memory Usage of all the keys in Redis DB
With no further ado, here is the script that uses some Shell commands and redis-cli
command-line tool
#!/bin/bash # To Find Redis TTL and Memory Usage # Author: Sarav AK REDISHOST=my-redis-broker.0001.use1.cache.amazonaws.com REDISPORT=6379 DBNO=4 KEYSLIST=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO keys "*"|awk '{print $1}'` echo "KEY TTL MEMORY_USAGE" echo "----------------------------- – – – – --" while read LINE do TTL=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO ttl "$LINE"`; MEMUSAGE=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO memory usage "$LINE"`; echo "$LINE $TTL $MEMUSAGE"; done <<< "$KEYSLIST"
Prerequisites
- You need to have the
redis-cli
tool installed in before executing this shell script - Also Necessary access to Redis
How it works
- It first lists all the keys by connecting to Redis database using the provided connection information
KEYSLIST=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO keys "*"|awk '{print $1}'`
- It passes the output of the previous command stored in
KEYSLIST
variable to a while loop where the following tasks are executed against each key-
- Get the TTL ( Time to Live) value of each key using
ttl
command - Get the Memory Usage value of each key using
memory usage
command
- Get the TTL ( Time to Live) value of each key using
while read LINE do TTL=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO ttl "$LINE"`; MEMUSAGE=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO memory usage "$LINE"`; echo "$LINE $TTL $MEMUSAGE"; done <<< "$KEYSLIST"
-
Where this can be used
This script can be used for All Redis deployments like Clustered or standalone
Can also be used in AWS Elastic Cache or any Managed Redis as well.
How to execute this script
Just copy the script and save it as *.sh
file and make sure it has executable permission by giving
chmod a+x findmemttl-redis.sh
I have named my script as findmemttl-redis.sh
you can rename it as you want
Now you can run the script
./findmemttl-redis.ssh
If you want some nice formatting and the values in separate columns. Execute the script with some awk like this
./findmemttl-redis.sh|awk '{ printf "%-50s %6s %s\n", $1, $2, $3 }'
If you have coloumn
install you can use that instead of awk
How to write the output as CSV
If you want to write the output as CSV, All you have to do is to change the spaces to commas in the following line
echo "$LINE $TTL $MEMUSAGE";
Here is the modified script which writes the output as CSV ( Comma Separated Values)
#!/bin/bash # To Find Redis TTL and Memory Usage # REDISHOST=my-broker-redis.use1.cache.amazonaws.com REDISPORT=6379 DBNO=4 KEYSLIST=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO keys "*"|awk '{print $1}'` echo "KEY,TTL,MEMORY_USAGE" while read LINE do TTL=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO ttl "$LINE"`; MEMUSAGE=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO memory usage "$LINE"`; echo "$LINE,$TTL,$MEMUSAGE"; done <<< "$KEYSLIST"
You can run the script with output redirection to .csv
file
./findmemttl-redis.ssh > output.csv
How to find the Non-Expiring Keys Redis
Sometimes you would not want all the keys, You might want only the Keys which do not have TTL configured.
In other words, Keys with no TTL set, The Non Expiring ones.
You can do that by adding a quick if condition into our script
#!/bin/bash # To Find Redis TTL and Memory Usage # REDISHOST=my-redis-broker.use1.cache.amazonaws.com REDISPORT=6379 DBNO=4 KEYSLIST=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO keys "*"|awk '{print $1}'` echo "KEY TTL MEMORY_USAGE" echo "----------------------------- – – – – -------------" while read LINE do TTL=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO ttl "$LINE"`; MEMUSAGE=`redis-cli -h $REDISHOST -p $REDISPORT -n $DBNO memory usage "$LINE"`; if [ $TTL -eq "-1" ] then echo "$LINE $TTL $MEMUSAGE"; fi done <<< "$KEYSLIST"
Here we have added an IF
statement to filter and print only the items which has a TTL
value as -1
TTL -1
represents that the key is non-expiring or has no TTL value set for that key.
Cheers
Sarav AK
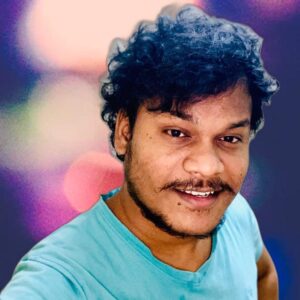
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content