Are you looking for a Shell Script that connects to remote server and executes some commands or another script? Are you looking for a Shell Script which SCP files to a remote server? Are you looking for Shell Script to SSH with Password and Automatically handle the Password Prompt?
Wondering how to manage the Password Prompt while using SSH and SCP inside the Script. ?
Else, Are you looking for an answer to any of the following questions
- SSH from Shell Script to Remote Server and Execute Multiple Commands
- shell script ssh with password
- shell script ssh without password prompt
- how to handle password prompt in a shell script
- ssh without key pair (or) key authentication
- SCP shell script with password
- SCP command in shell script without prompting password
Then this post is for you
So how do you connect to remote Linux server Normally ( From Terminal)
To Login to remote servers we use SSH
and to transfer files between Linux Servers we SCP
. I presume that you might have used this in your experience.
Now while using the SSH and SCP command you would be prompted for the password before it lets you do anything with the remote Linux Server.
If it is terminal you can actually enter/type the password yourself and proceed.
But what will you do when you want to use the SSH and SCP commands inside the Shell Script.
So, How do you handle the Password Prompt of SSH and SCP inside the Shell Script?
There are two ways.
- Create Passwordless SSH connection between servers using Key-based Authentication
- Use SSHPass to pass the password with your SCP and SSH commands. discussed in this post
Yes the objective of this post is to make you able to use SSH and SCP inside the Shell Script and handling the Password prompt without having to create Key based authentication
So let's Proceed.
Before going to the Shell Script. Let us see the same requirement done in the terminal/shell.
So, How to connect to SSH or SCP without being prompted for the Password (Terminal)
The Answer is. You should use SSHPASS along with your SSH and SCP commands
What!!!.
SSHPASS command reads the password from a FILE or from STDIN and pass it on to the SSH and SCP command, and thereby preventing the SSH and SCP commands from prompting for a password
See the example in real time.
So as shown in the preceding record. I have to perform the following steps to log in to the remote server without being prompted or in other words, Make SSH read password from a file using SSHpass
Step1: Create a password file and type in your password as a clear text ( Not a Secure Method )
# Write the password into a file and Save it
[vagrant@mwivmapp01 ~]$ cat > .passwrdfile
vagrant
# Display the content of the file
[vagrant@mwivmapp01 ~]$ cat .passwrdfile
vagrant
Step2: Refer the password file in SSHPASS and pass it to SSH.
# Logging into mwivmapp02 from mwivmapp02 using SSHPASS and SSH
[vagrant@mwivmapp01 ~]$ sshpass -f.passwrdfile ssh mwivmapp02
Last login: Sat Jun 1 20:36:14 2019 from 192.168.43.11
[vagrant@mwivmapp02 ~]$ exit
[vagrant@mwivmapp01 ~]$
In the preceding snippet shown. you can find that there was no password prompt and we have successfully logged in to the remote server [mwivmapp02
]
There is a Security flaw in this approach. Whoever gets access to this password file can get the password as it is a plain text. therefore, it is not a recommended approach.
How to use SSHPASS inside the Shell Script ( A Secure Approach )
Now we are going to use the SSHPASS inside the Shell Script and this time we are going to read the password from the user instead of keeping it in a file
we are going to use sshpass -p
for that. Here -p represents the Clear Text password
We cannot use this in the terminal as the history would show the password as a clear text to whoever logged in to the System.
But with Script it is OK as the Variables are alive only during the lifetime of the Script and they cannot be seen in the history.
The Script does the following tasks
- Gets UserName and Password from the User
- Read the list of server names from a
Serverlist.properties
file - Create a Script on the Runtime named
TestScript.sh
using HereDocument - Copy the Created TestScript to the remote server using SCP
- Execute the Copied TestScript on the remote server using SSH
The Serverlist.properties file
we have intentionally kept only one server. you can have more based on your need.
# cat Serverlist.properties
mwivmapp02
The Script file [RemoteExec.sh]
#!/bin/bash
# Author: Sarav AK - [email protected]
# Date: 2 June 2019
#
#
# Get the UserName to use while logging into a Remote machine
echo "Enter the Remote UserName"
read rmtuname
echo "Enter the Remote Password"
read -s rmtpasswrd
# Read the ServerNames from Properties file
for server in `cat Serverlist.properties`
do
# Printing the ServerName
echo "Processing ServerName "$server
# Write some Shell Script for Temporary Usage and Save in Current location
cat << 'EOF' > ./TestScript.sh
#!/bin/bash
echo "My Name is $0"
echo "I am Running on `hostname`"
echo "The Date on the Current System is `date`"
echo "That's all!!. I am Exitting"
exit 0
EOF
chmod a+x TestScript.sh
# SCP - copy the script file from Current Directory to Remote Server
sshpass -p$rmtpasswrd scp -o UserKnownHostsFile=/dev/null -o StrictHostKeyChecking=no TestScript.sh $rmtuname@$server:/tmp/TestScript.sh
# Take Rest for 5 Seconds
sleep 5
# SSH to remote Server and Execute a Command [ Invoke the Script ]
sshpass -p$rmtpasswrd ssh -o UserKnownHostsFile=/dev/null -o StrictHostKeyChecking=no $rmtuname@$server "/tmp/TestScript.sh"
done
The Dynamically Created TestScript.sh
#!/bin/bash
echo "My Name is $0"
echo "I am Running on `hostname`"
echo "The Date on the Current System is `date`"
echo "That's all!!. I am Exitting"
exit 0
Runtime Output of RemoteExec.sh
[root@mwivmapp01 tmp]# hostname
mwivmapp01
[root@mwivmapp01 tmp]# ./RemoteExec.sh
Enter the Remote UserName
vagrant
Enter the Remote Password
Processing ServerName mwivmapp02
Warning: Permanently added 'mwivmapp02,192.168.43.12' (ECDSA) to the list of known hosts.
Warning: Permanently added 'mwivmapp02,192.168.43.12' (ECDSA) to the list of known hosts.
My Name is /tmp/TestScript.sh
I am Running on mwivmapp02
The Date on the Current System is Sat Jun 1 22:17:07 UTC 2019
That's all!!. I am Exitting
[root@mwivmapp01 tmp]#
You can see that the script has been created dynamically and shared with the remote server and executed and the output has been displayed.
Some Security Testing I did, to verify if ps reveals my password
I wanted to see if this is a Real Secure approach
Being aware that, All the commands being executed inside the Shell Script would eventually show up in the result of PS at least during the time of execution
Though the sshpass -p
is inside the script. I thought if another user who have access to the terminal can use ps command to get the password
So I wanted it to test it myself.
I used ps -auxwww
command and was searching for the keyword sshpass on the mwivmapp01
server after invoking the script
This is what I got.
I found some Random Characters replacing my actual password. Thanks to the Developers of SSHPASS
So It is clear that you cannot get the password using PS using this Method. Hence it is proved to be Secure
Hope this article helps. Rate this article [ratings]
If you find any bug in this article (or) security issues with this approache please feel free to enlighten me
Thanks,
Sarav AK
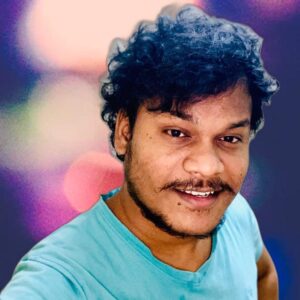
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content