Ansible APT Package manager is an Ubuntu equivalent for RedHat yum package manager. Just like all other ansible modules apt
ansible module is built after one specific unix command of Debian apt-get
It is always recommended to choose the modules rather using the raw unix commands over the shell module as it would bring more standard and fault tolerance to your Ansible Playbook
So how to use ansible apt module in the playbook or as an ad hoc.
Well!. this article is going to cover ansible apt module in detail with various examples.
Introduction to ansible apt module
Ansible apt module manages apt packages in Debian or Ubuntu systems.
In typical Ubuntu machine, in order to install a package, you would execute the following command
apt install nginx
Same way here in ansible, the following playbook/play would do that for you
---
- name: Playbook to install NGINX
hosts: webservers
become: true
tasks:
- name: Ansible apt install nginx
apt:
name: nginx
state: present
Here is the quick screen snip of executing this playbook.
The Difference between apt and apt-get
Some of us including me would have most often referred ubuntu apt (aptitude) as apt-get
we use both apt
and apt-get
interchangeably
The following statement from Debian blog would help us understand the difference between apt and apt-get
apt
is a second command-line based front end provided by APT which overcomes some design mistakes ofapt-get
.
Based on this, You can understand that the apt module is fairly advanced than its predecessor apt-get
So apt could do everything and more what apt-get could possibly do.
As far as Ansible apt module is concerned, it uses the apt command over apt-get and it could fall over to apt-get automatically in some cases where necessary.
But, What If I want ansible to use apt-get over apt?
Well Ansible apt module supports apt-get commands as well and you could use instruct ansible apt module to use apt-get using a parameter named force_apt_get
The preceding playbook example can be rewritten like this with force_apt_get
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible apt-get to get install nginx
apt:
name: nginx
state: present
force_apt_get: yes
The Ansible apt Examples
We have collected some of the interesting and useful examples of Ansible apt module here.
How to install a Package with Ansible apt
We have already seen an example of how to install a package with ansible apt in the introduction.
However, Here is one more playbook example that installs a Single Package or software named apache2
---
- name: Playbook to install Apache
hosts: webservers
become: true
tasks:
- name: Ansible apt install Apache
apt:
name: apache2
state: present
here the state: present
would tell ansible to make it available and installed.
How to remove a Package with Ansible apt
To remove a package with Ansible apt. All you have to do use state: absent
along with the package name of your choice.
Let us use the same playbook we have used for installation for example here with a little modification
---
- name: Playbook to install Apache
hosts: webservers
become: true
tasks:
- name: Ansible apt install Apache
apt:
name: apache2
state: absent
You can notice that all we had to change is the state
How to do an apt update with Ansible
In this section, we are going to see how to update the cache with ansible apt
It is always recommended and a kind of best practice all the administrators would follow while installing a package. Just to make sure that they are getting the updated package from the Source and not the cached one on the local server.
In order to update the local cache, we have to use update_cache
parameter in our playbook
To put it in a perspective. Let's suppose we want to install the latest OpenSSL version in our ubuntu server. these are the command we would execute in our server
apt update apt install openssl (or) apt-get update apt-get install openssl
With an ansible apt module, we can do the same by just adding update_cache
parameter. Here is the playbook with update_cache which installs the latest version OpenSSL
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible Update Cache and install openssl
apt:
name: openssl
state: present
update_cache: yes
Update cache if it is older than a certain time
Sometimes updating the cache may not be necessary at all as it would have been updated only a few minutes or days before.
So we can instruct Ansible to update the cache only if the current cache is older than a specific time. Mentioned in seconds.
here is the playbook which updates the cache if the current cache is built 3600 seconds(1 hour) ago
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible apt update cache if it is older than 1 hour
register: updatesys
apt:
update_cache: yes
cache_valid_time: 3600
Upgrade a Single package or Software with ansible apt
Let us suppose we want to upgrade the OpenSSL version installed in our system and this is the command we would execute on our servers
# Update the Cache apt update # Upgrade the package openssl apt upgrade openssl (or) apt-get update apt-get upgrade openssl
With ansible apt module this is how it can be done
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible Update Cache and upgrade openssl
apt:
name: openssl
state: latest
update_cache: yes
If you have not noticed it already. the state: latest
parameter would make sure that the package is upgraded and stay the latest.
this is how we upgrade the package or tool with an ansible apt module.
Update & Upgrade All the packages installed in OneGo
It is always recommended to upgrade the packages installed in our system to maintain system security and integrity.
In Enterprises, this would be done every quarterly or even monthly during the scheduled patching.
So how to update & upgrade all the packages or tools installed Debian/ubuntu
Differentiate the Update and Upgrade
The update is to update all the packages in the local repository, It is also known as updating the cache
The upgrade is to upgrade the packages installed on the system, you can think of this one as more of a Version Upgrade.
These are the Linux commands we would execute in order to achieve this
# Update the Cache apt update # Upgrade all the packages installed apt upgrade (or) apt-get update apt-get upgrade
here is the ansible-playbook to update and upgrade the packages in ubuntu
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible Update Cache and Upgrade all Packages
register: updatesys
apt:
name: "*"
state: latest
update_cache: yes
- name: Display the last line of the previous task to check the stats
debug:
msg: "{{updatesys.stdout_lines|last}}"
This is the output our playbook would yield in already updated and up-to-date system
You can notice that the last line is displaying the statistics of the packages upgraded, installed etc.
How to install a list of packages in One Go with Ansible apt
So we have been so far installing a Single Package but when it comes to real-time server provisioning we would be installing N number of packages based on our requirements.
So it is not wise to create plays for each package installation like we have been so far doing.
The right approach to install multiple packages with ansible apt is to use ansible loops and iteration methods. A Simple Programming paradigm
This is the playbook we are going to use to install / setup a LAMP (LinuxApacheMysqlPhp) stack on our Debian/Ubuntu server
- name: Ansible apt module examples hosts: web become: yes tasks: - name: Ansible apt to install multiple packages - LAMP register: updatesys apt: update_cache: yes name: - firewalld - apache2 - mariadb-server - php - php-mysql state: present
Here is the execution result of this playbook with Debug mode on
Install Specific version of a package with Ansible apt
Sometimes we would want to install a very specific version of a package or software for various reasons.
let's see how to specify the package version with ansible apt module.
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible apt install tomcat Version 8.0.32
register: updatesys
apt:
update_cache: yes
name: tomcat8=8.0.32-1ubuntu1.11
state: present
The preceding playbook helps to install the tomcat version 8.0.32
Install a .deb
file or package using ansible apt
ansible apt module can be used to install the .deb packages as well additionally ansible apt can download the file from remote URL and install it as well.
So here is the playbook where we are trying to install a *.deb
package. But the Source of these deb files are different.
One is already downloaded and kept in /tmp directory and another one is yet to be downloaded from the URL
As mentioned earlier, Ansible apt can download the package automatically from the remote URL and install it without any manual intervention.
here we are going to install filebeat using the deb file.
---
- name: Ansible apt module examples
hosts: web
become: true
tasks:
- name: Ansible install filebeat deb file from local
apt:
deb: /tmp/filebeat-7.6.0-amd64.deb
- name: Ansible install filebeat deb file from url
apt:
deb: https://artifacts.elastic.co/downloads/beats/filebeat/filebeat-7.6.0-amd64.deb
How to validate if a package is installed - Ansible apt
Sometimes you would want to validate if the package is installed or not without making any modifications to the system
Though it can be done with Ansible apt module and check_mode
I would recommend using package_facts
module to validate if the package is installed or not.
here is the sample playbook to validate if few packages are installed or not.
---
- name: Ansible validate if the packages are installed
hosts: web
become: true
tasks:
- name: Gather Package facts
package_facts:
manager: auto
- name: Validating if the package is installed or not
debug:
msg: "{{item}} is installed"
when: '"{{item}}" in ansible_facts.packages'
with_items:
- apache2
- nginx
- filebeat
Here is the output of this playbook.
You can see that it prints only apache2 is installed, as the other two are not installed they were skipped
Clean up the useless Packages using ansible apt
To Clean up the useless packages from the system you can use ansible apt module like shown below.
It clears the useless packages from cache and remove the dependency tools which are no longer required.
- name: Remove useless packages from the cache
apt:
autoclean: yes
- name: Remove dependencies that are no longer required
apt:
autoremove: yes
Conclusion
Hope the given examples of Ansible apt module helps you understand how to use ansible apt module for managing Debian packages.
Cheers
Sarav AK
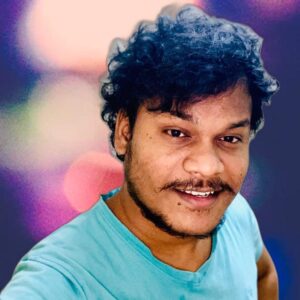
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content