In this article, we are going to see how to use ansible pre tasks and post tasks with examples.
What is Ansible pre_tasks?
Ansible pretask is a conditional execution block that runs before running the play. It can be a task with some prerequisites check (or) validation
Some examples we can think where ansible pre-task would be helpful
- To install dependency packages before running the actual application
npm install
orpip install -r requirements.txt
- To validate if the environment meets sufficient criteria like memory, OS version etc before installing a software
- configuring SSH key prior so that you can log in
- Server provisioning steps like creating user, group etc before the installation or setup begin
There are more examples we can continue adding to this list
To put it simply. If you want to run anything as a prerequisite or prevalidation before your actual play. ansible pre_tasks is your way to go
What is Ansible post_tasks?
Just like ansible pre_tasks but this is executed after the actual play or task is completed.
this is mostly useful for post validation or assertion to make sure things are in the right shape or the result is matching our expectations.
Some examples we can think of are listed below
- To validate if things were installed properly and the playbook executed fine.
- To send emails or Slack notifications after successful completion of a playbook
- Running some other task or for accessing some API or external service upon playbook completion like webhook
- Starting the application after the installation and configuration is completed
We can keep this list going with more and more use cases for ansible post_tasks
Simply put, ansible post_tasks is to conditionally execute a block or play upon the successful completion of the playbook. it can be used for post validation, post-execution automation.
How to use pre_tasks and post_tasks in Ansible
Let us see how to use ansible pre_tasks
and post_tasks
in the ansible-playbook practically.
In this playbook, we are going to perform the following tasks
-
- Install necessary commands and tools using
apt
module -pre_tasks
- Validate the nodejs installation is successful and print version using debug and assert
- Create a directory to download the nodejs application
- Download the nodejs codebase from GIT repo using Tokenized URL
- Tokens are saved and retrieved from the Secrets file named
gitsecrets.yml
- Do
npm install
once the code is downloaded - Start the nodejs application
- Validate if the port is open and Node js is accepting requests
- Send Slack notification using ansible slack module -
post_tasks
- Install necessary commands and tools using
--- - name: Install and Launch the Simple NodeJS Application hosts: testserver vars_files: - gitsecrets.yml vars: - destdir: /apps/sampleapp pre_tasks: - name : install dependencies before starting become: yes register: aptinstall apt: name: - nodejs - npm - git state: latest update_cache: yes - name : validate the nodejs installation debug: msg="Installation of node is Successfull" when: aptinstall is changed tasks: - name: Version of Node and NPM shell: "npm -v && nodejs -v" register: versioninfo - name: Validate if the installation is intact assert: that: "versioninfo is changed" - name: Create Dest Directory if not exists become: yes file: path: "{{ destdir }}" state: directory owner: vagrant group: vagrant mode: 0755 - name: Download the NodeJS code from the GitRepo become: yes git: repo: 'https://{{gittoken}}@github.com/AKSarav/SampleNodeApp.git' dest: "{{ destdir }}" - name: Change the ownership of the directory become: yes file: path: "{{destdir}}" owner: "vagrant" register: chgrpout - name: Install Dependencies with NPM install command shell: "npm install" args: chdir: "{{ destdir }}" register: npminstlout - name: Debug npm install command debug: msg='{{npminstlout.stdout_lines}}' - name: Start the App async: 10 poll: 0 shell: "(node index.js > nodesrv.log 2>&1 &)" args: chdir: "{{ destdir }}" register: appstart - name: Validating the port is open tags: nodevalidate wait_for: host: "localhost" port: 5000 delay: 10 timeout: 30 state: started msg: "NodeJS server is not running" post_tasks: - name: notify Slack that the servers have been updated tags: slack community.general.slack: token: T026******PF/B02U*****N/WOa7r**********Ao0jnWn msg: | ### StatusUpdate ### – ------------------------------------ `` `Server`: {{ansible_host}} `Status`: NodeJS Sample Application installed successfully – ------------------------------------ channel: '#ansible' color: good username: 'Ansible on {{ inventory_hostname }}' link_names: 0 parse: 'none' delegate_to: localhost
You can see how we are using pre_tasks
and post_tasks
in this playbook
we are using pre_tasks
to run a task that installs necessary components and packages like node, npm etc
Learn more about, how to use apt with ansible.
and using post_tasks
to send a notification to the Slack channel upon Task completion using the ansible slack module and webhook. I will try to cover this slack integration in detail in my next article
Here is the output of this playbook
$ ansible-playbook install-nodejs-app.yaml -i hosts – ask-vault-password Vault password: PLAY [Install and Launch the Simple NodeJS Application] ************************************************************************************ TASK [Gathering Facts] ********************************************************************************************************************* ok: [testserver] TASK [install dependencies before starting] ************************************************************************************************ changed: [testserver] TASK [validate the nodejs installation] **************************************************************************************************** ok: [testserver] => { "msg": "Installation of node is Successfull" } TASK [Version of Node and NPM] ************************************************************************************************************* changed: [testserver] TASK [Validate if the installation is intact] ********************************************************************************************** ok: [testserver] => { "changed": false, "msg": "All assertions passed" } TASK [Create Dest Directory if not exists] ************************************************************************************************* changed: [testserver] TASK [Download the NodeJS code from the GitRepo] ******************************************************************************************* changed: [testserver] TASK [Change the ownership of the directory] *********************************************************************************************** ok: [testserver] TASK [Install Dependencies with NPM install command] *************************************************************************************** changed: [testserver] TASK [Debug npm install command] *********************************************************************************************************** ok: [testserver] => { "msg": [ "[email protected] /apps/sampleapp", "├─┬ [email protected] ", "│ ├─┬ [email protected] ", "│ │ ├─┬ [email protected] ", "│ │ │ └── [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ ├── [email protected] ", "│ │ ├─┬ [email protected] ", "│ │ │ ├── [email protected] ", "│ │ │ └── [email protected] ", "│ │ ├─┬ [email protected] ", "│ │ │ └── [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ ├── [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ ├── [email protected] ", "│ │ ├── [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├── [email protected] ", "│ ├─┬ [email protected] ", "│ │ └── [email protected] ", "│ ├── [email protected] ", "│ └── [email protected] ", "└─┬ [email protected] ", " ├── @node-redis/[email protected] ", " ├─┬ @node-redis/[email protected] ", " │ ├── [email protected] ", " │ ├── [email protected] ", " │ ├─┬ [email protected] ", " │ │ └── [email protected] ", " │ └── [email protected] ", " ├── @node-redis/[email protected] ", " ├── @node-redis/[email protected] ", " └── @node-redis/[email protected] " ] } TASK [Start the App] *********************************************************************************************************************** changed: [testserver] TASK [Validating the port is open] ********************************************************************************************************* ok: [testserver] TASK [notify Slack that the servers have been updated] ************************************************************************************* ok: [testserver -> localhost] PLAY RECAP ********************************************************************************************************************************* testserver : ok=13 changed=6 unreachable=0 failed=0 skipped=0 rescued=0 ignored=0
Conclusion
In this article, we have learnt what is ansible pre tasks and ansible post tasks and how to use them.
As a summary, we learnt pre_tasks
is to execute some tasks prior/before executing the actual play or tasks. there can be many tasks under pre_tasks
Likewise, ansible post_tasks
is to execute some tasks post/after the successful completion of the actual play or tasks. Like sending emails and slack notifications etc.
We have also learned how to send slack notifications using ansible post_task in this article along with a few more tricks like
- Ansible Assertion
- Ansible Git
- How to use ansible wait_for
- how to install packages with Ansible apt
- Ansible Shell.
- How to use Ansible Vault and secrets in the playbook
We have around 50+ articles dedicatedly for each of these modules. in DevopsJunction.
Please refer to the following article and make use of it.
Ansible Playbook Examples – Sample Ansible Playbooks | Devops Junction
Cheers
Sarav AK
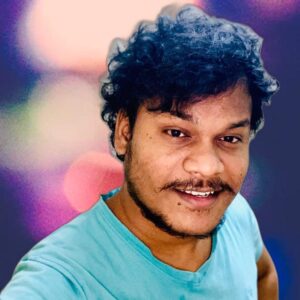
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content