Ansible SystemD module helps to control the systemd units such as services and timers created on the Linux server.
SystemD is a daemon that manages the services and timer units on the Linux system and we mostly interact with it using the following ways
- service file
- systemctl command
- journalctl command
- timer unit files
Linux Engineers mostly use it for managing the services but it have timer
feature which works like a CRON and helps in scheduling a task
If you are working on Linux, there are high chances you might have used any of these commands to interact with the services.
service command
service <someservice> status service <someservice> start service <someservice> reload service <someservice> restart
systemctl command
systemctl status <someservice> systemctl start <someservice> systemctl reload <someservice> systemctl restart <someservice>
In this article, we are going to see how to manage services and service unit files on Linux using Ansible
So if you are entirely new to Linux services and have no background. Please refer to this article and learn what is systemd
If you are entirely new to Ansible. please start with our Ansible beginner Articles
Now let us shift our focus to our objective, the Ansible SystemD module
Quick Example of Ansible SystemD - Restarting a Service
Here is a quick example of an Ansible SystemD module to restart an nginx service
--- - name: Ansible SystemD Example hosts: testserver tasks: - name: restart NGINX service become: true systemd: name: nginx state: restarted
Let us see what each field means in this playbook
- hosts - host or hostgroup name where the task to be executed
- tasks
- name - name of the task(play)
- become - to execute the task with sudo privilege as root user
- systemd - module name
- name - service name
- state - action to be taken on the service ( restarted | reloaded | started | stopped )
here is the execution result of this playbook
to validate if the nginx service is actually restarted. you can use the following ad hoc command
ansible testserver -m shell -a "systemctl status nginx"
Stop the service using ansible systemd
Here is the ansible playbook to stop the service using the ansible systemd module
--- - name: Ansible SystemD Example hosts: testserver tasks: - name: stop NGINX service become: true systemd: name: nginx state: stopped
here is the output of this playbook when executed
$ ansible-playbook SystemD-examples.yaml -i hosts PLAY [Ansible SystemD Example] ************************************************************************************************ TASK [Gathering Facts] ******************************************************************************************************** ok: [testserver] TASK [stop NGINX service] ***************************************************************************************************** changed: [testserver] PLAY RECAP ***************************************************************************************************************** testserver : ok=2 changed=1 unreachable=0 failed=0 skipped=0 rescued=0 ignored=0
Start the service using ansible systemd
Here is the playbook we can use to start the nginx service using the ansible systemd module
--- - name: Ansible SystemD Example hosts: testserver tasks: - name: Start NGINX service become: true systemd: name: nginx state: started
Here is the output of this playbook as a screen record
Reload the service using ansible systemd
we have already seen how to restart the service using the ansible systemd module earlier. here is how we can reload the service using
Especially, services like nginx often reloaded but not restarted. reload would not kill the existing connections or transactions managed by the service but just reload the configuration.
Let us see how to reload a service using the ansible systemd module
this is more like performing systemctl reload nginx
command in real-time
Here is the playbook to reload the service in ansible
--- - name: Ansible SystemD Example reload service hosts: testserver tasks: - name: reload NGINX service become: true systemd: name: nginx state: reloaded
Here is the output of this playbook when I executed it on my end
Enable the service using ansible systemd
Creating a unit file for any application/software makes the administration easier. But there is one more primary requirement we do have.
It is to configure the services automatically during the boot time ( start time).
Nowadays most of the installations do come up with the creation of services and enabling at boot by default.
To check if your service is enabled to run at boot we can use the systemctl show command
systemctl show <servicename>|grep -i UnitfileState
we often use systemctl enable command to enable services to run at boot time
systemctl enable <servicename.service>
Now let us see how to enable a service with an ansible systemd module.
Here is the playbook
--- - name: Ansible SystemD Example hosts: testserver tasks: - name: Enable NGINX service become: true systemd: name: nginx enabled: yes
Here is the output when executed from our end. I have added some additional validation ad hoc commands too
You can see from the screenshot that the service is enabled and we are validating it with an ansible ad hoc command
In case if you want to disable a service using ansible systemd. you can use the same playbook above but change the
enabled:
tono
Creating a service/systemd unit file with ansible
we have so far learnt how to manage the existing unit files or services with the ansible systemd module.
Now let us see how to create a service unit file with ansible.
Those who have created systemd unit files aka service files already in Linux know that it is a little complex as it has a lot of features to offer.
We do not have any dedicated module in Ansible to create these unit files for us.
the SystemD module also limits just managing the unit files so we need to create the unit files ourselves.
So here is some real-time example playbook where we install and set up a tomcat webserver and create a service for it.
This playbook performs the following tasks
- Create a group and user named
tomcat
- Create a directory named
/opt/tomcat8
- Download Tomcat and unarchive
- move the files to the right directory
- Create the service file in /etc/systemd/system
- reload the systemd
- enable and start the tomcat service
- validate by accessing the tomcat webapp URL
this playbook is tested.
--- - name: Installation and Setup of Tomcat8 hosts: testserver tasks: - name: Create the group become: yes group: name: tomcat state: present - name: Create the user become: yes user: name: tomcat state: present - name: Create a Directory /opt/tomcat8 become: yes file: path: /opt/tomcat8 state: directory mode: 0755 owner: tomcat group: tomcat - name: Download Tomcat using unarchive tags: never become: yes unarchive: src: https://dlcdn.apache.org/tomcat/tomcat-8/v8.5.81/bin/apache-tomcat-8.5.81.zip dest: /opt/tomcat8 mode: 0755 remote_src: yes group: tomcat owner: tomcat - name: Move files to the /opt/tomcat8 directory tags: never become: yes become_user: tomcat shell: "mv /opt/tomcat8/apache*/* /opt/tomcat8" - name: Creating a service file become: yes copy: dest: /etc/systemd/system/tomcat.service content: | [Unit] Description=Tomcat Service Requires=network.target After=network.target [Service] Type=forking User=tomcat Environment="CATALINA_PID=/opt/tomcat8/logs/tomcat.pid" Environment="CATALINA_BASE=/opt/tomcat8" Environment="CATALINA_HOME=/opt/tomcat8" Environment="CATALINA_OPTS=-Xms512M -Xmx1024M -server -XX:+UseParallelGC" ExecStart=/opt/tomcat8/bin/startup.sh ExecStop=/opt/tomcat8/bin/shutdown.sh Restart=on-abnormal [Install] WantedBy=multi-user.target - name: Reload the SystemD to re-read configurations become: yes systemd: daemon-reload: yes - name: Enable the tomcat service and start become: yes systemd: name: tomcat enabled: yes state: started - name: Connect to Tomcat app on port 8080 and check status 200 uri: url: http://localhost:8080/
In this complete playbook, we have two tasks that match our objective
Reloading the systemd Daemon using an ansible systemd module
- name: Reload the SystemD to re-read configurations become: yes systemd: daemon-reload: yes
The next one is to enable and start the service using the systemd module
- name: Enable the tomcat service and start become: yes systemd: name: tomcat enabled: yes state: started
Conclusion
Hope you have learnt how to use the ansible systemd module and I hope all the examples are making sense.
If you have any questions. do let us know in the comments section.
Cheers
Sarav AK
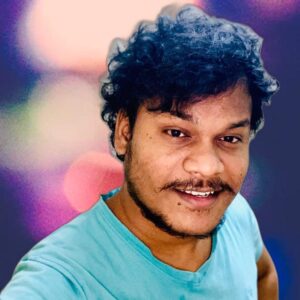
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content