This is a Simple Python Flask Application to Upload files in various formats like CSV, XLS, XLSX, PDF, JPG, PNG, GIF, TXT and save those files into the File System
The Application is Designed with a Simple interface to Upload a file in any of those aforementioned formats. The Supported file extensions or formats can be modified within the code.
The Uploaded files would be saved into a directory named upload_dir
on the current directory where the Flask Application Code is placed and started. This Location can be modified to suit your needs.
The upload_dir
would be created if it is not already present. The Actual Saving location would be something like upload_dir/08061910
Where 08 - Current Date, 06 - Current Month, 19 - Current Year, 10 - Current Hour
It will create a new directory for every new hour (only when the file is being uploaded). If two or multiple files are uploaded at the same hour the same directory would be used.
Here is the Sample Screen Shot of our End Result.
With no further ado. Here is the code.
Python Flask Code to Upload a File
Here is the Flask Application Code. Save this file as FileCollect.py
import os
import datetime
import subprocess
from flask import Flask, flash, request, redirect, url_for
from werkzeug.utils import secure_filename
app = Flask(__name__)
app.secret_key = b'_5#y2L"F4Q8z\n\xec]iasdfffsd/'
ALLOWED_EXTENSIONS = set(['txt', 'pdf', 'png', 'jpg', 'jpeg', 'gif', 'csv'])
def CreateNewDir():
print "I am being called"
global UPLOAD_FOLDER
print UPLOAD_FOLDER
UPLOAD_FOLDER = UPLOAD_FOLDER+datetime.datetime.now().strftime("%d%m%y%H")
cmd="mkdir -p %s && ls -lrt %s"%(UPLOAD_FOLDER,UPLOAD_FOLDER)
output = subprocess.Popen([cmd], shell=True, stdout = subprocess.PIPE).communicate()[0]
if "total 0" in output:
print "Success: Created Directory %s"%(UPLOAD_FOLDER)
else:
print "Failure: Failed to Create a Directory (or) Directory already Exists",UPLOAD_FOLDER
def allowed_file(filename):
return '.' in filename and \
filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
@app.route('/', methods=['GET', 'POST'])
def upload_file():
if request.method == 'POST':
# check if the post request has the file part
if 'file' not in request.files:
flash('No file part')
return redirect(request.url)
file = request.files['file']
# if user does not select file, browser also
# submit an empty part without filename
if file.filename == '':
flash('No selected file')
return redirect(request.url)
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
UPLOAD_FOLDER = './upload_dir/'
CreateNewDir()
global UPLOAD_FOLDER
file.save(os.path.join(UPLOAD_FOLDER, filename))
return redirect(url_for('uploaded_file',
filename=filename))
return '''
<!doctype html>
<title>Upload new File</title>
<h1>Upload new File</h1>
<form method=post enctype=multipart/form-data>
<input type=file name=file>
<input type=submit value=Upload>
</form>
'''
@app.route('/uploaded', methods=['GET', 'POST'])
def uploaded_file():
return '''
<!doctype html>
<title>Uploaded the file</title>
<h1> File has been Successfully Uploaded </h1>
'''
if __name__ == '__main__':
app.secret_key = 'super secret key'
app.config['SESSION_TYPE'] = 'filesystem'
sess.init_app(app)
app.debug = True
app.run()
Start and Stop Flask Server and Application
In General, These three steps are enough to start the Flask Application.
$ export FLASK_APP=FileCollect.py
$ export FLASK_ENV=development
$ flask run
But Its not THE Efficient way. So I built some Wrapper Shell Script around this to Start and Stop my Flask Server and Application.
The Shell Script - Flask-Manage.sh
Here is the Shell Script to Stop and Start the Flask Application. It reads the necessary Startup parameters like
- What Action to Implement?
- What is the Python file?
- What is the Startup Environment {PROD|DEV}?
#!/bin/bash
BASEDIR=`dirname $0`
Action=$1
Infile=$2
Environment=$3
function startserver
{
export FLASK_ENV=$Environment
export FLASK_APP=$Infile
#flask run – host=0.0.0.0 – port=80
flask run – host=0.0.0.0 – port=5000
}
function stopserver
{
ps -eaf|grep -i "flask run"|grep -iv grep|awk '{print $2}'|xargs kill -9
}
if [ $Action == "Start" -o $Action == "start" -o $Action == "START" ]
then
echo "Starting the Server"
startserver > $BASEDIR/flask.log 2>&1 &
exit
elif [ "$Action" == "Stop" -o "$Action" == "stop" -o "$Action" == "STOP" ]
then
echo "Stopping the Server"
stopserver >> $BASEDIR/flask.log
fi
To Start the Server in Development Environment use the following command
./Flask-Manage.sh START FileCollect.py DEV
To Start the Server in Production Environment
./Flask-Manage.sh START FileCollect.py PROD
To Stop the Running Server use the following command [ Kills all locally running Flask run processes ]
./Flask-Manage.sh STOP
The Log File
On the Same Directory where the Code and Flask-Manage Script resides. there would be flask.log
file containing the Access and Debug logs of your Flask Application and Server.
* Serving Flask app "FileCollect.py"
* Environment: DEV
* Debug mode: off
* Running on http://0.0.0.0:5000/ (Press CTRL+C to quit)
Command Snippet Record - Demo Video
Here is the Video Demo of using this Flask Upload Application.
Hope this helps.
Rate this article [ratings]
Thanks,
Sarav AK
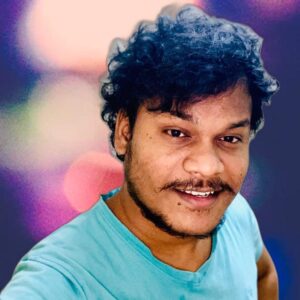
Follow me on Linkedin My Profile Follow DevopsJunction onFacebook orTwitter For more practical videos and tutorials. Subscribe to our channel

Signup for Exclusive "Subscriber-only" Content